Day 22: Mastering Docker Building Images
Mar 7, 2025
•11:26 AM GMT+8
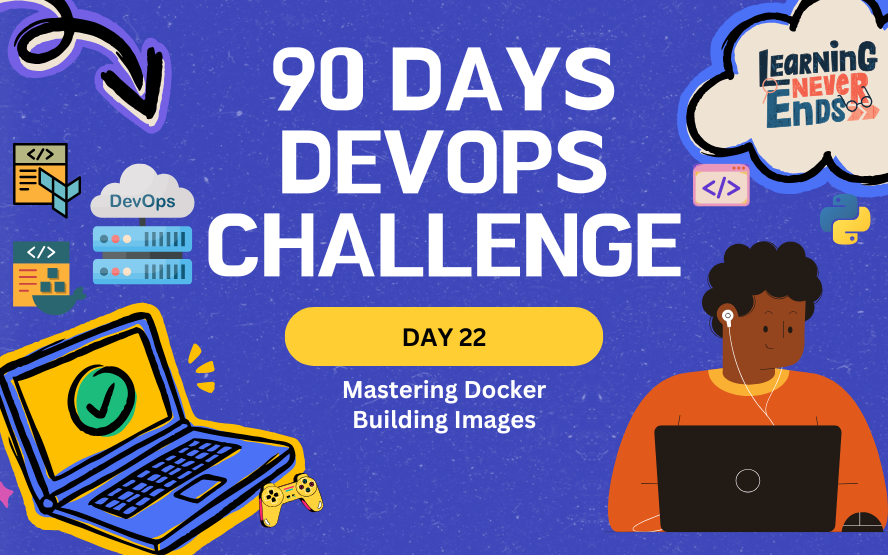
Using Docker images, applications can be packaged with their dependencies for seamless execution across different environments. The Dockerfile, an automates the process of creating images, contains the requirements for the application, dependencies, and configurations. The purpose of this blog is to cover the basics of building images, understanding Dockerfile instructions, and implementing a practical task by containerizing a Django web application from our GitHub repository.
Build Images
The Docker image is a lightweight, standalone, and executable package that includes all the files needed to run an application, including the code, runtime, libraries, and dependencies. A Docker image is immutable, which means that once built, it cannot be altered or modified. Only new images can be created using Docker.
What is Dockerfile?
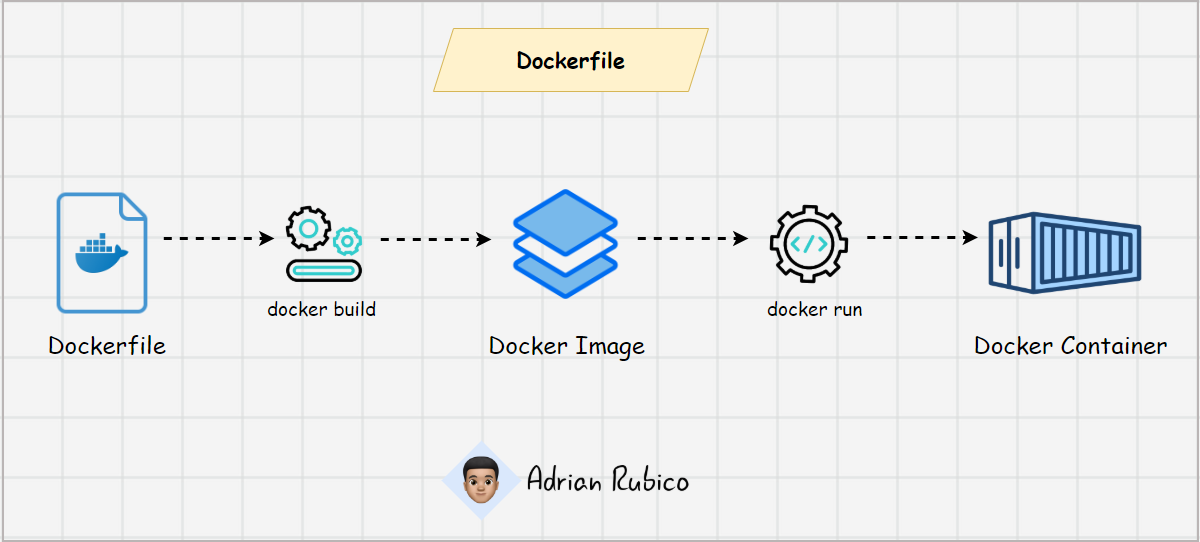
A Dockerfile is a text document containing instructions for creating a Docker image. It defines how the container should be built, what dependencies to install, and how to execute the application inside the container.
Dockerfile Instructions
The following table summarizes key Dockerfile instructions:
Instruction | Description |
FROM | Specifies the Base image. |
WORKDIR | Set the working directory inside the container. |
COPY | Only copies files and directories from the source to the destination. |
ADD | Can copy files/directories and automatically extract compressed archives. |
RUN | Executes commands during the build process. |
ENV | Defines environment variables inside the container. |
VOLUME | Creates a mount point and marks it as holding externally mounted volumes. |
EXPOSE | Container listens on the specified network ports at runtime. |
CMD | Provides default commands and/or arguments for running container. |
ENTRYPOINT | It defines the primary command that will always be executed when the container starts. |
Tasks
Build a Web Application Image
For this task, we will build a Docker image for a Django web application using the repository DjangoCrudAjax.
Steps:
- Clone the repository:
git clone https://github.com/git-adrianrubico/DjangoCrudAjax.git
- Create Dockerfile:
# Use the official Python 3.14 slim base image
FROM python:3.14-rc-slim
# Create a directory named 'app' inside the container
RUN mkdir /app
# Set the working directory to '/app'
WORKDIR /app
# Set environment variables to optimize Python behavior
# Prevents Python from writing .pyc files (bytecode)
ENV PYTHONDONTWRITEBYTECODE=1
# Ensures logs are output immediately instead of being buffered
ENV PYTHONUNBUFFERED=1
# Copy all project files from the host to the '/app' directory inside the container
COPY . /app/
# Install project dependencies listed in 'requirements.txt'
RUN pip install --no-cache-dir -r requirements.txt
# Expose port 8000 to allow external access to the application
EXPOSE 8000
# Define the command to start the Django development server
CMD ["python", "manage.py", "runserver", "0.0.0.0:8000"]
The file structure would look like the following:
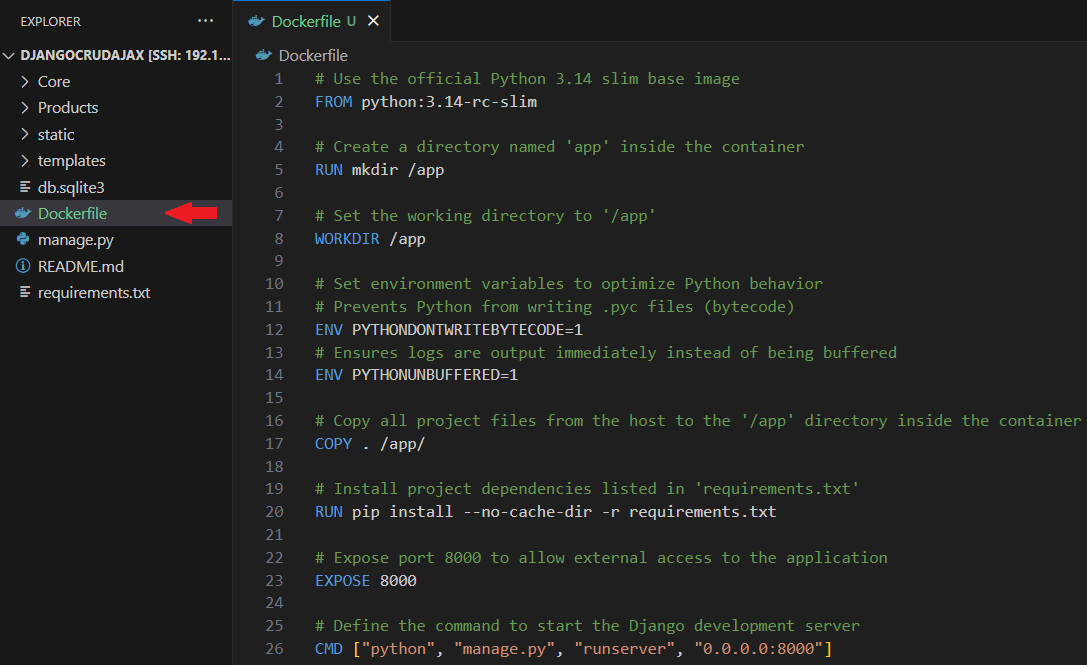
- Build the Docker image:
docker build -t django-crud-app:v1 .
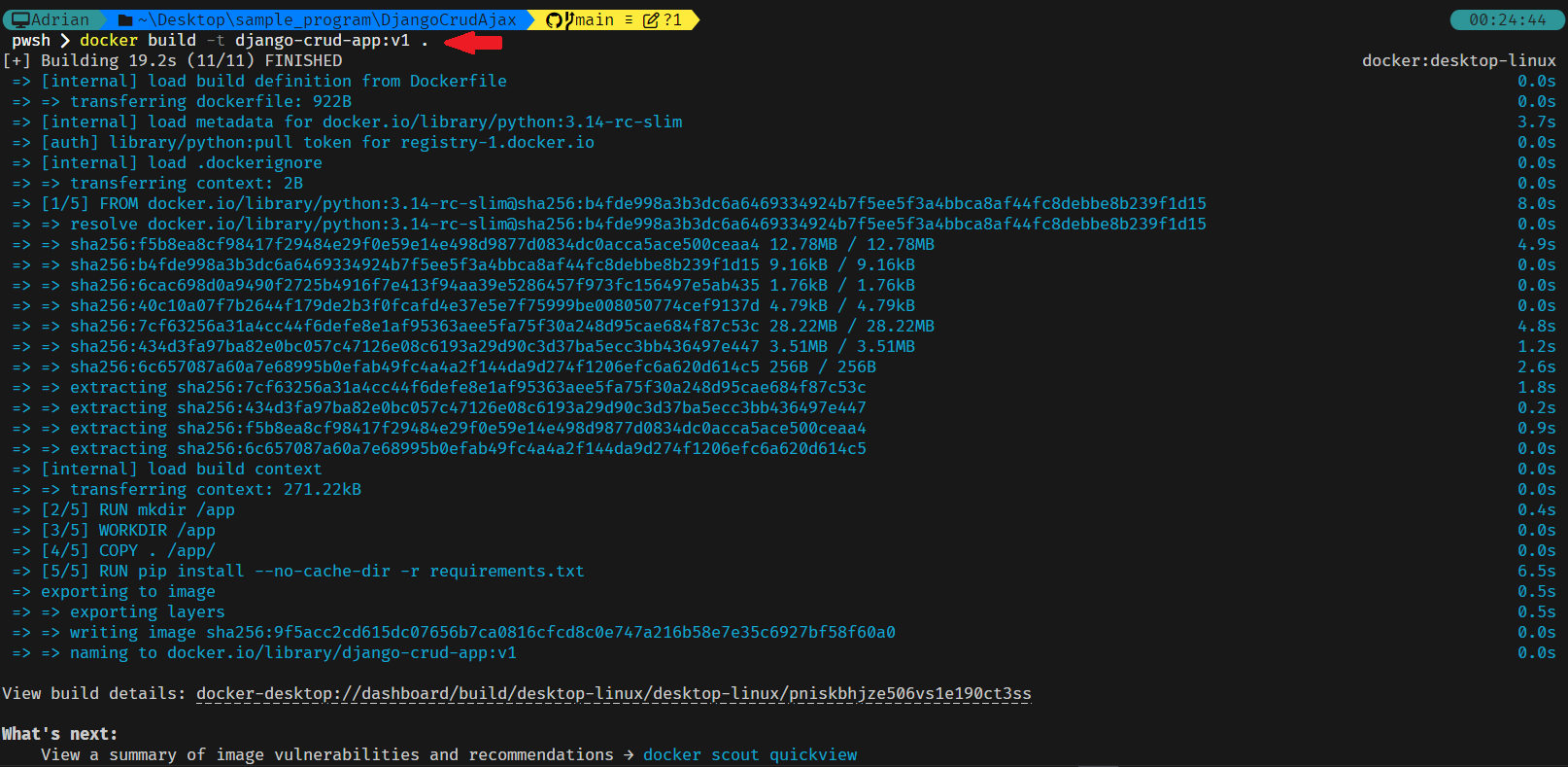
- Run the container:
docker run -d -p 8000:8000 --name django-crud-app django-crud-app:v1

- Access the web application in the browser at
http://localhost:8000
.
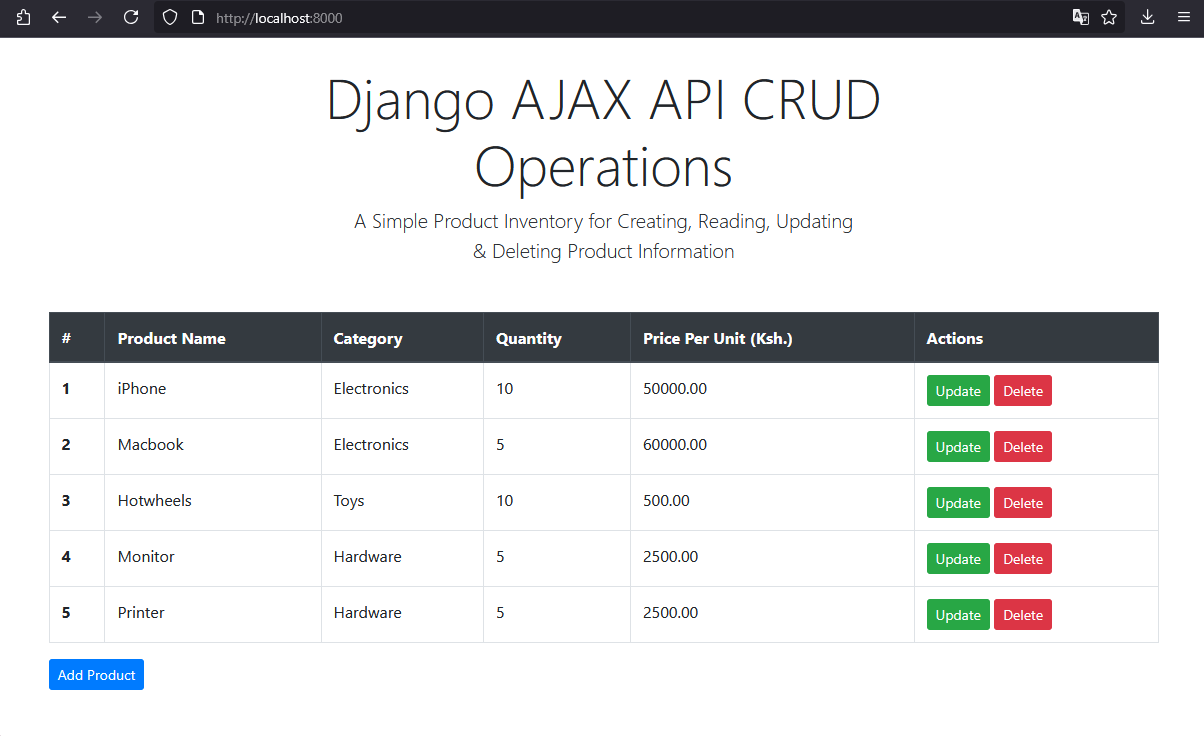
Host the Built Image on Docker Hub
Once the image is built, we can push it to Docker Hub to share and deploy it across different environments.
Steps:
- Login to Docker Hub:
docker login

- Tag the image (Replace
your-username
with your Docker Hub username):
docker tag django-crud-app your-username/django-crud-app:v1

- Push the image to Docker Hub:
docker push your-username/django-crud-app:v1
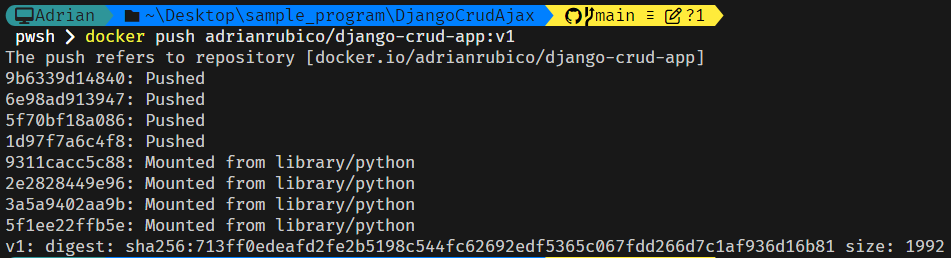
- Verify the image is available on Docker Hub by visiting your profile at hub.docker.com:
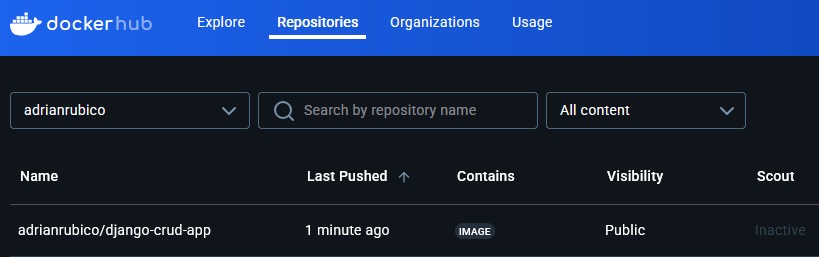
- Pull and run the image from any machine (In my case I test it with my Linux VM):
# Pull the Image
docker pull your-username/django-crud-app:v1
# Run the image
docker run -p 8000:8000 your-username/django-crud-app:v1
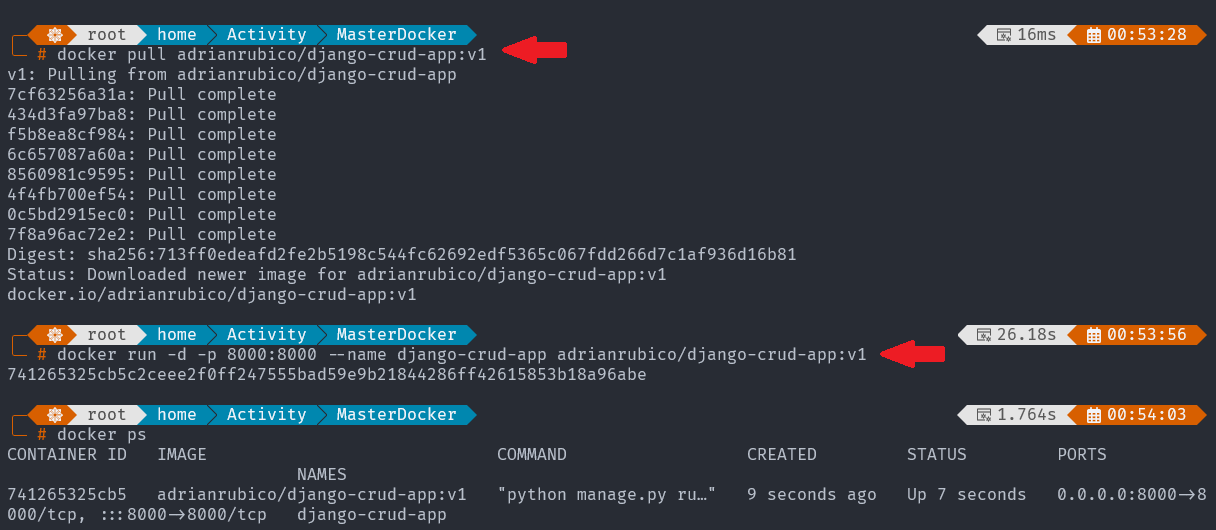
Conclusion
As part of this blog, we discussed building Docker images, Dockerfiles, and Dockerfile instructions. Additionally, we containerized a Django web application as a practical exercise.
Next, we will learn about the differences and best practices between Dockerfile instructions Entrypoints and CMD.
Day 22: Mastering Docker Building Images
Learn how to build Docker images, write Dockerfiles, containerize a Django app, and push images to Docker Hub.
For the passion of automated cloud solutions.
Subscribe to get the latest posts. I mostly write about Backend (Python/Bash), DevOps and Linux.