Day 4: Mastering Bash Scripting - Variables
Aug 10, 2024
•07:04 PM GMT+8
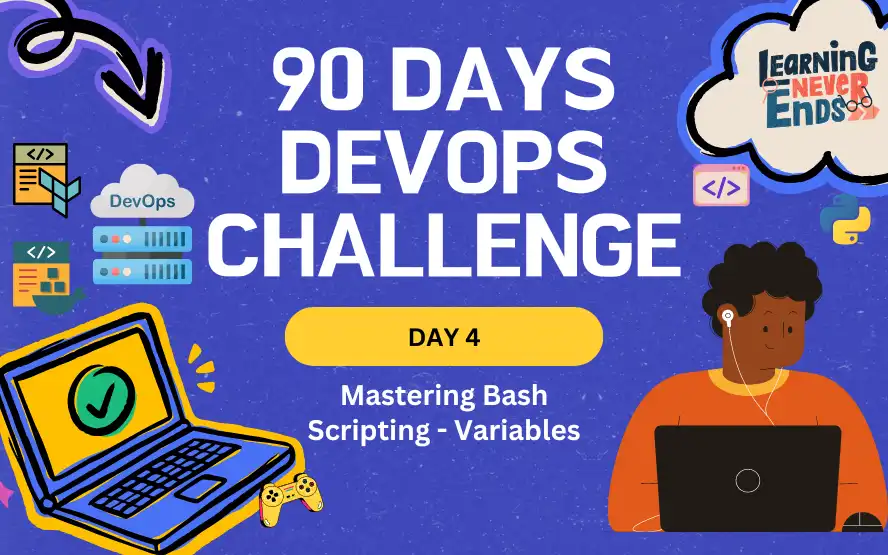
Previously, we covered the basics of Bash scripting, which involved creating our initial script and understanding the Linux kernel and shell. As we proceed, we will focusing on variables in Bash scripts. Through these concepts, we can create dynamic scripts capable of storing and manipulating data.
Understanding Variables
Variables are temporary storage locations in memory. They are essentially placeholders that can hold different types of data, such as strings, numbers, or even the output of commands. Since variables hold information temporarily, there are two key actions we can perform on them.
- Assigning a value to a variable
- Accessing or utilizing the value to a variable
Here is how you can assign a variables in Linux terminal. In this example, variable VAR1
is assigned the value #90DaysDevOpsChallenge
. To access the value of a varaible, you prefix it with a dollar sign ($
).
VAR1=#90DaysDevOpsChallenge
echo $VAR1
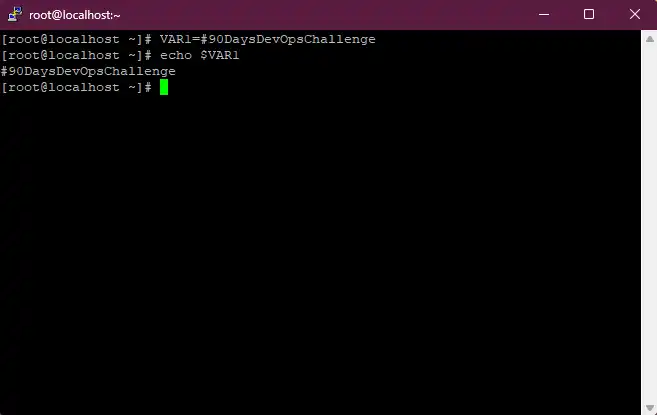
We can also apply this in our Bash script file.
#!/bin/bash
# Assign a value to a variable
VAR1="#90DaysDevopsChallenge"
# Use the variable
echo $VAR1
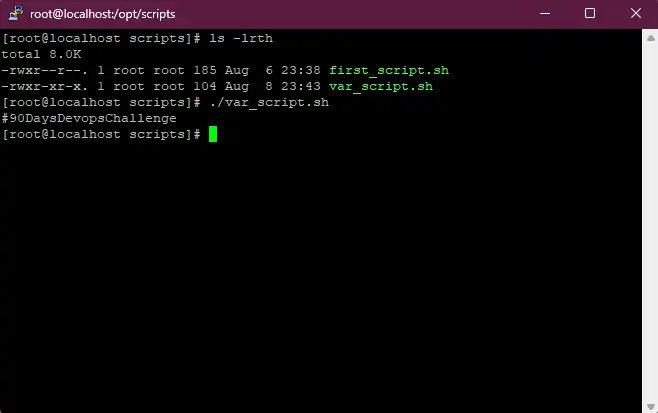
Variables can also store the results of commands
#!/bin/bash
# Assign a value to a variable
VAR1="#90DaysDevopsChallenge"
# Store the current data in a variable
CURRENT_DATE=$(date)
# Use the variable
echo $VAR1
echo "----------------------"
# Output the current date
echo "Today's date is : $CURRENT_DATE"
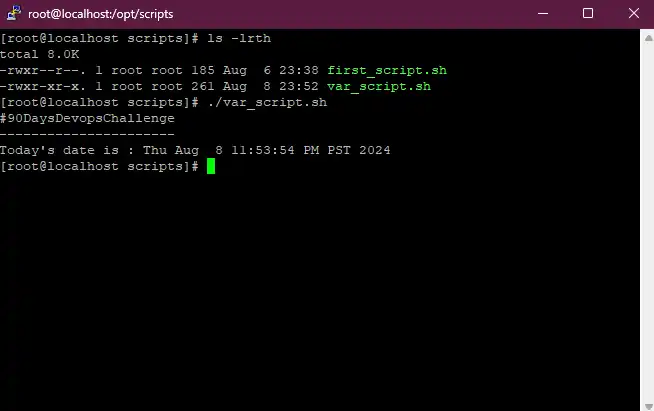
Command-Line Arguments in Variables
Along with predefined variables, Bash scripts can accept command-line arguments. By using these arguments, users can pass data directly to the script when they execute it, making the script more interactive and resourceful.
For example, consider a script that accepts a user’s name as a command-line argument
#!/bin/bash
# Assign the first command-line argument to a variable
name=$1
# Greet the User
echo "Hello, $name!"
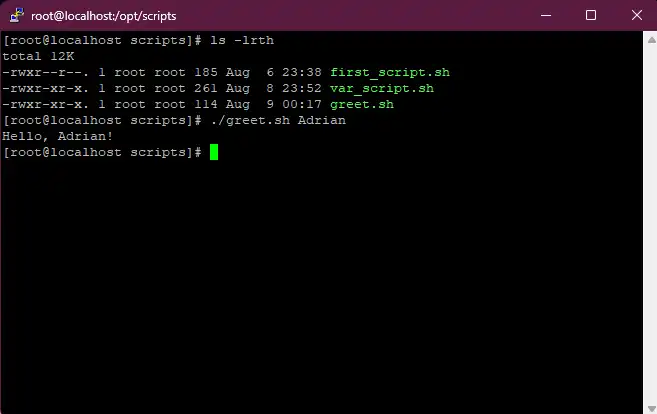
In this script, $1
refers to the first command-line argument provided when running the script. If you run the script with the command ./greet.sh Adrian
, the output will be Hello, Adrian!
.
We can use system commands with multiple command-line arguments with this script.
#!/bin/bash
# Make multiple Folder
mkdir $1 $2 $3
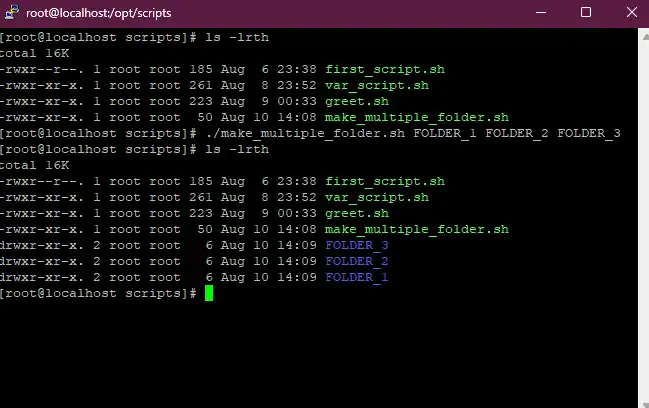
System Variables
We can use system variables that already set in the Linux environment
System Variable | Description |
$0 | The name of the script itself |
$1, $2, ... | The first, second, ... command-line arguments passed to the script |
$# | The number of command-line arguments passed to the script |
$@ | All command-line arguments as a single string |
$$ | The process ID (PID) of the current script |
$? | The exit status of the last executed command |
$USER | The identity of the user executing the script |
$HOSTNAME | The hostname of the machine where the script is executed |
Understanding Quotes & Command Substitution
Bash scripting uses quotes to control how variables and special symbols are interpreted.
There are three types of quotes you can use
- Double Quotes (
"
) - Single Quotes (
'
) - Backticks (
`
)
Double Quotes ("
): Variables and special characters within double quotes are evaluated and expanded.
NAME="Adrian"
echo "Congratulations $NAME, you won \$1 million dollars"
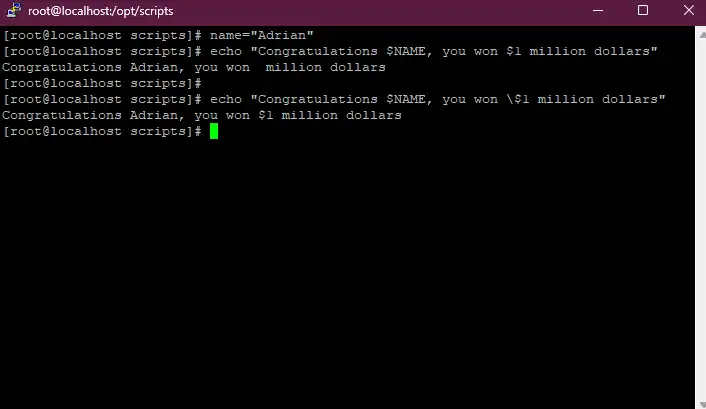
In this example, the $NAME
variable is expanded to its value, "Adrian," and the dollar sign before "1 million dollars" is escaped using \
, so it’s treated as a literal character.
Single Quotes ('
): Everything inside single quotes is taken literally, and no variable expansion occurs.
NAME="Adrian"
echo 'Congratulations $NAME, you won $1 million dollars'
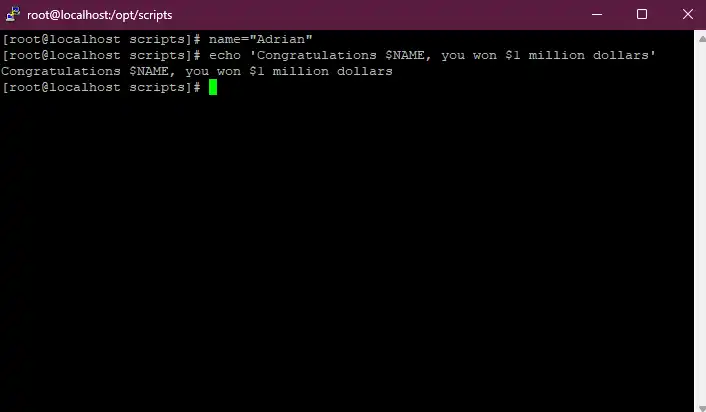
Here, the text inside the single quotes is not expanded, so $NAME
and $1
are treated as plain text, not variables.
Backticks (`
): Used for command substitution, where the output of a command is inserted in place of the command itself.
current_date=`date`
echo "Today's date is: $current_date"
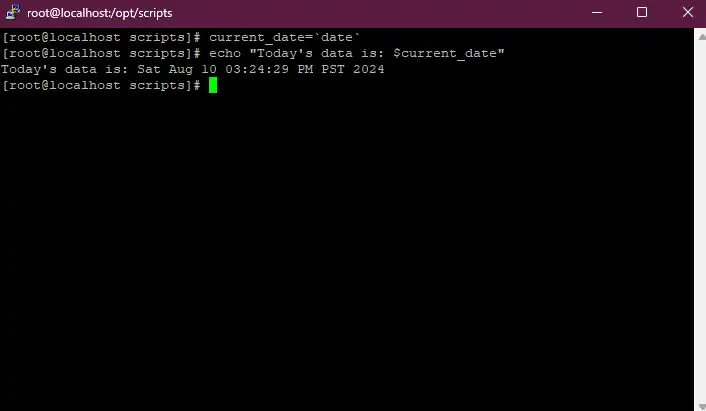
Variables
By default, variables in Bash are only available in the shell in which they were created. In some cases, you may want to make a variable available to child processes or other scripts. This is where exporting variables comes in.
# CLI
$ VARNAME="Adrian"
$ export VARNAME
# test_export_01.sh / test_export_02.sh
#!/bin/bash
echo "Hello $VARNAME you executed script $0"
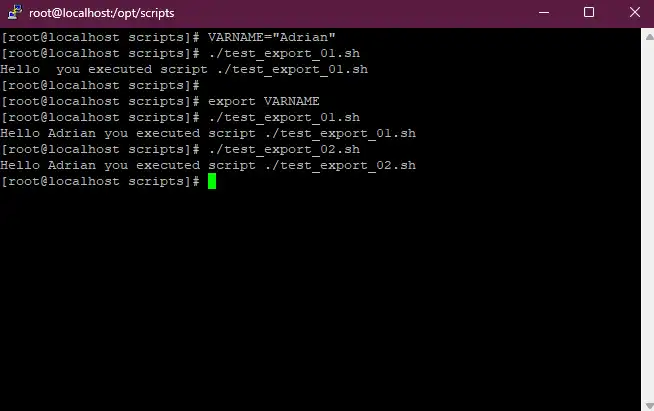
In this example, the VARNAME
variable is exported, making it accessible to test_export.sh
. Without the export
command, the VARNAME
variable would not be available outside of the script or shell where it was defined.
💡 It is important to remember that exporting variables in this way is temporary. Variables will only be available to child processes started from the current shell session. When you close the terminal or start a new session, the exported variables are lost.
Make variables Permanently
If you want to make a variable permanent across all sessions, you need to add it to a configuration file like ~/.bashrc
, ~/.bash_profile
or /etc/profile
(for login shells). Here’s how you can do it:
- Open the
~/.bashrc
,~/.bash_profile
or/etc/profile
file in your favorite text editor
vi ~./bashsrc
- Add your variable, export it, save the file, and exit the editor.
export VARNAME="Adrian"
- To apply the changes immediately, source the file
export VARNAME="Adrian"
Looking Ahead
Scripts are created by manipulating and using variables, which form the foundation of Bash scripting. Mastering this concept is essential for writing effective scripts, whether by using simple user-defined variables, command-line arguments, system variables, or exporting variables to other scripts. In addition, understanding the proper use of quotes ensures that your scripts interpret and process variables and text correctly.
In the next section of this series, we will delve into conditions in Bash scripting. This will allow you to make decisions and execute code based on specific criteria. Stay tuned for more insights on mastering Bash scripting!
Day 4: Mastering Bash Scripting - Variables
Find out how to use variables in Bash scripts, along with command-line arguments, system variables, quotes, command substitution, and exporting variables.
For the passion of automated cloud solutions.
Subscribe to get the latest posts. I mostly write about Backend (Python/Bash), DevOps and Linux.