Day 5: Mastering Bash Scripting - Conditions
Aug 11, 2024
•08:34 PM GMT+8
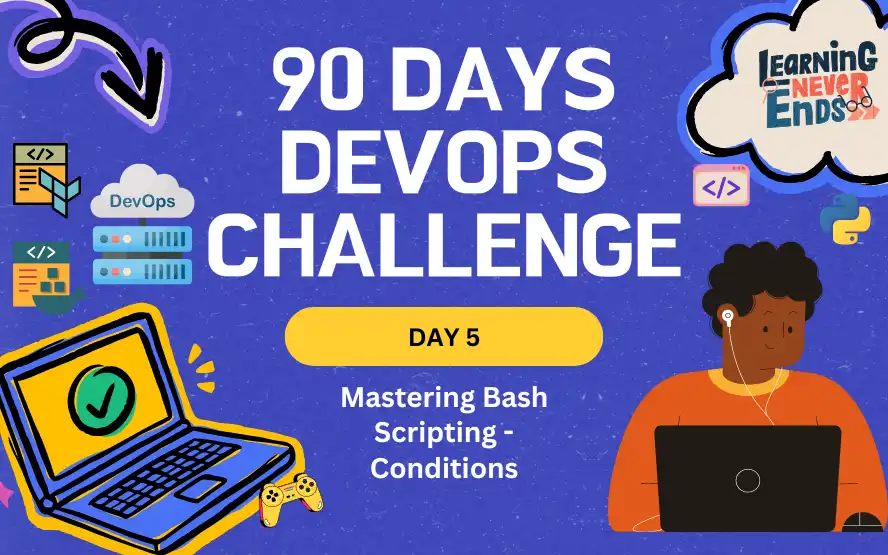
In our last lesson, we learned about variables in Bash scripts. Now, we are going to dive into conditions. Conditions let your scripts make choices and do different things based on what's happening, making them more flexible and useful.
User Inputs
Before we jump into conditions, it's crucial to understand how to handle user input in your Bash scripts. User input allows your scripts to interact with users by asking for information or making choices during execution.
#!/bin/bash
read -p "Please enter your name: " name
echo "Hello, $name!"
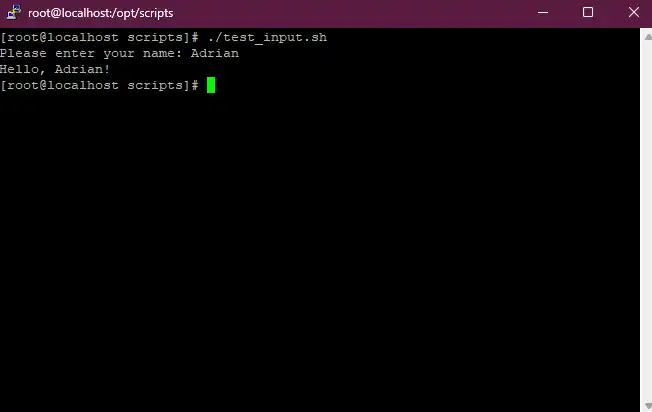
The -p
option allows you to specify a prompt message, making the interaction more intuitive for the user.
Understanding Conditions
In Bash, conditions control the flow of script execution. It evaluates expressions to determine if they are true or false, allowing scripts to make decisions accordingly. This fundamental aspect is essential for creating dynamic and responsive Bash programs.
The most common way to implement conditions in Bash is through the use of if
, elif
, and else
statements. Here’s the basic syntax.
if [ condition ]; then
# Code to execute if condition is true
else
if [ another_condition ]; then
# Code to execute if the second condition is true
else
# Code to execute if none of the conditions are true
fi
fi
Simple if
Statements
Let’s start with a simple example of an if statement that checks whether a file exists
#!/bin/bash
file="first_script.sh"
if [ -e "$file" ]; then
echo "The file '$file' exists."
else
echo "The file '$file' does not exist."
fi
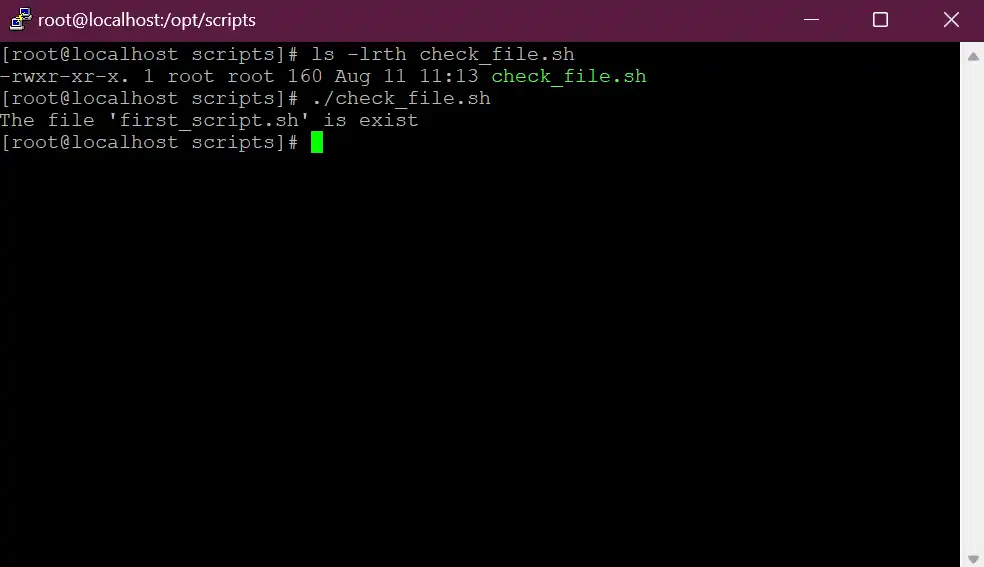
In this script, the -e
option checks if the file exists. If the condition is true, the script prints a message saying the file exists. Otherwise, it prints a message saying the file does not exist.
Using elif
for Multiple Conditions
The elif
keyword (short for "else if") allows you to check multiple conditions in sequence. Here’s an example
#!/bin/bash
read -p "Enter a number: " NUM
echo "--------------"
if [[ ! $NUM =~ ^[0-9]+$ ]]; then
echo "Please input numbers only"
elif [[ $NUM -gt 100 ]]; then
echo "The number is greater than 100"
elif [[ $NUM -lt 100 ]]; then
echo "The number is less than 100"
else
echo "The number is exactly 100"
fi
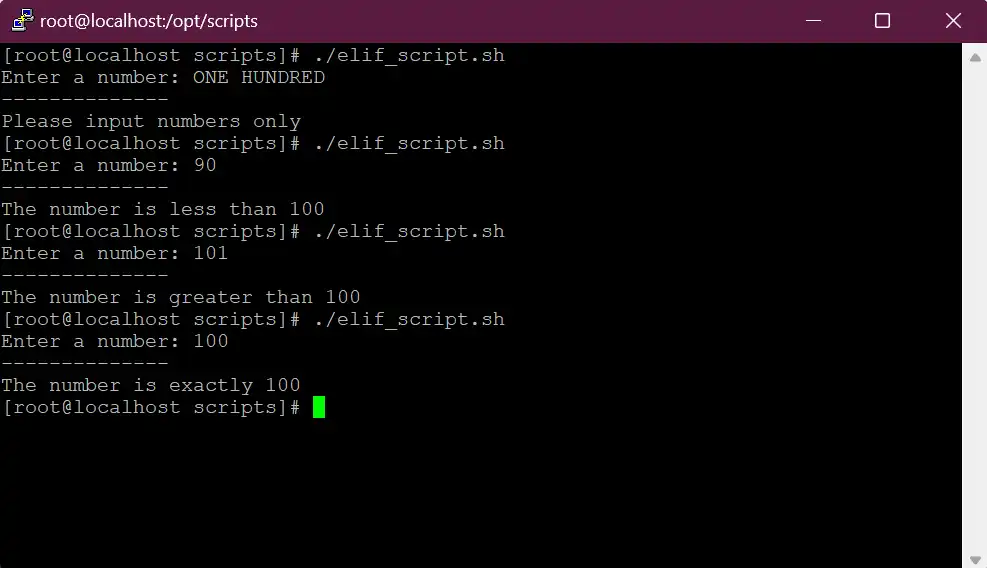
Lets break down the script based on the example above.
- User Input: The script prompts the user to enter a number, storing it in the variable
NUM
. - Input Validation: The condition
[[ ! $NUM =~ ^[0-9]+$ ]]
checks if the input contains only digits. If not, it prompts the user to input numbers only. Condition Checks:
- If the number is greater than 100, it outputs "The number is greater than 100."
- If less than 100, it outputs "The number is less than 100."
- If neither, it outputs "The number is exactly 100."
💡 You may check this Bash Cheatsheet for more example of Conditions Statements.
Wrapping Up
A condition is a powerful feature in Bash scripting that allows you to make decisions and control the flow of your scripts. No matter how you use if
statements or else if
statements for various scenarios, mastering conditions makes your scripts much more dynamic and responsive.
In the next part of this series, we will continue our exploration of Bash scripting by diving into loops. Loops will enable you to repeat tasks and iterate over data, adding another layer of functionality to your scripts. Stay tuned for more!
Day 5: Mastering Bash Scripting - Conditions
Explore the power of conditional logic in Bash scripting to automate decision-making processes based on user input and variable values.
For the passion of automated cloud solutions.
Subscribe to get the latest posts. I mostly write about Backend (Python/Bash), DevOps and Linux.