Day 6: Mastering Bash Scripting - Loops
Aug 14, 2024
•12:37 AM GMT+8
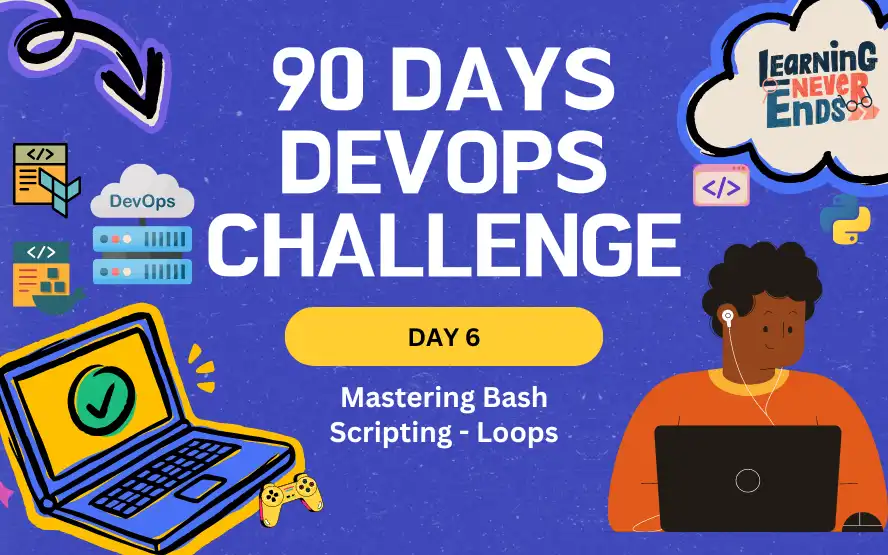
The use of loops in Bash scripting allows you to automate repetitive tasks effectively. In this part of our series, we'll explore the various types of loops in Bash and how to use them effectively.
Understanding Loops
Loops in Bash enable you to execute a block of code multiple times, depending on certain conditions. Bash supports several types of loops, each suited for different tasks:
for
Loop: Iterates over a list of items.while
Loop: Repeats a block of code as long as a condition is true.
Let's examine each of these loop types in detail.
The for
Loop
The for
loop is one of the most commonly used loops in Bash. It iterates over a list of items and executes a block of code for each item.
Example: Iterating Over a List of Folders
#!/bin/bash
for i in /opt/scripts/FOLDER_*; do
echo "Folder Name: $i"
done
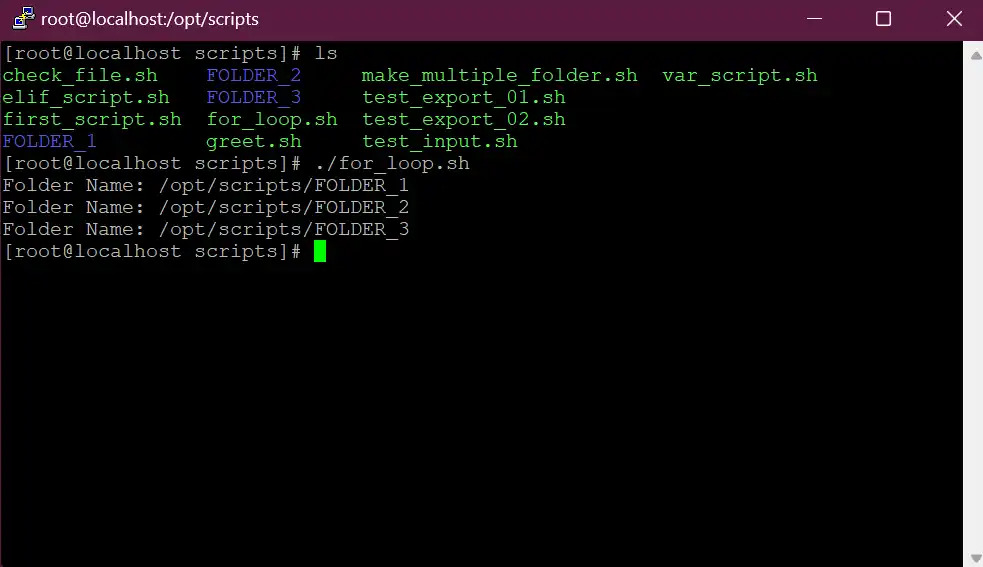
The for
loop iterates over all FOLDER_
folders in the current directory. For each file, it prints "Folder Name:" followed by the folder name. This is particularly useful for batch processing files or performing similar actions on multiple items.
The while
Loop
The while
loop continues to execute a block of code as long as a specified condition is true.
Countdown Timer
#!/bin/bash
COUNT=5
while [[ $COUNT -gt 0 ]]; do
echo "Countdown: $COUNT"
((COUNT--))
sleep 1
done
echo "Done while loop!"
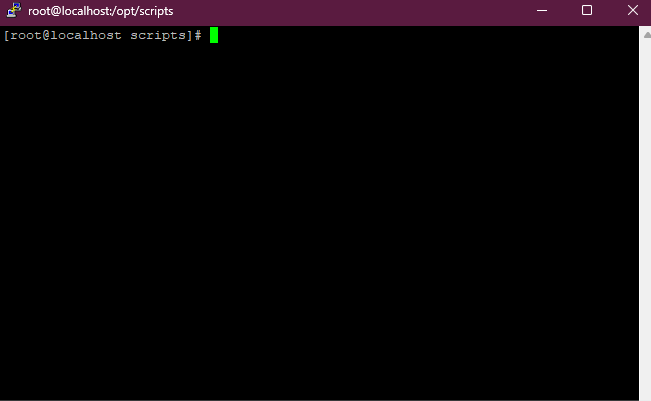
The loop starts with COUNT
set to 5 and decreases by 1 each iteration. The loop continues until COUNT
reaches 0, at which point it prints "Done while loop!
". This type of loop is ideal for scenarios where you need to wait for a condition to change, such as waiting for a process to complete or monitoring a system state.
Looking Ahead
In Bash scripts, loops enable you to automate repetitive tasks efficiently. Understanding loops and how to utilize them can make them more powerful, flexible, and effective.
In our next blog, we will explore the awk
command, a powerful tool for text processing and data extraction in Bash scripting. Stay tuned!
Day 6: Mastering Bash Scripting - Loops
Make repetitive tasks easier by learning the basics of for and while loops in Bash scripting.
For the passion of automated cloud solutions.
Subscribe to get the latest posts. I mostly write about Backend (Python/Bash), DevOps and Linux.