Day 25: Mastering Docker Multi-stage Builds
Mar 23, 2025
•09:40 PM GMT+8
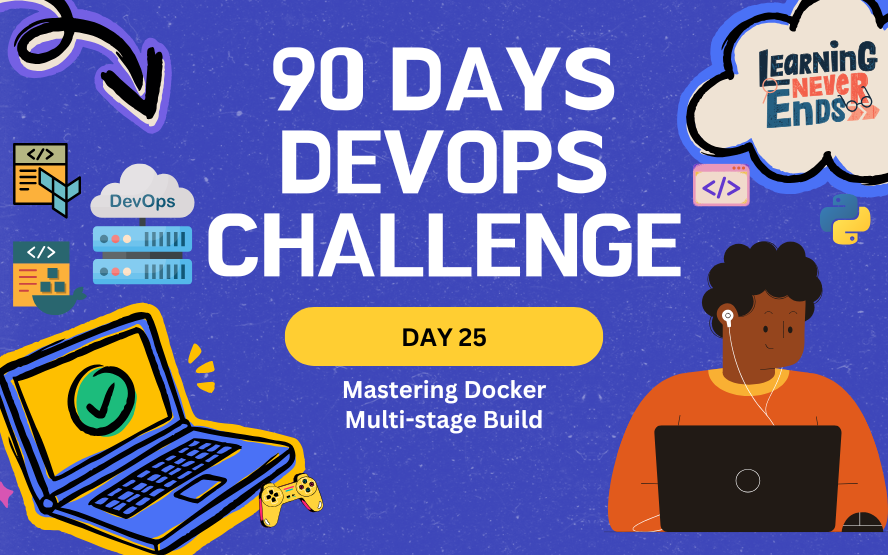
In our previous blog(Day 25: Mastering Docker Multi-stage Build), we containerized the DjangoCrudAjax application using Docker Compose with MySQL for data persistence. However, our current Dockerfile includes unnecessary dependencies that increase the image size.
With Docker, we will implement a multi-stage build, reducing the final image size and improving security and performance.
What is Multi-stage Build?
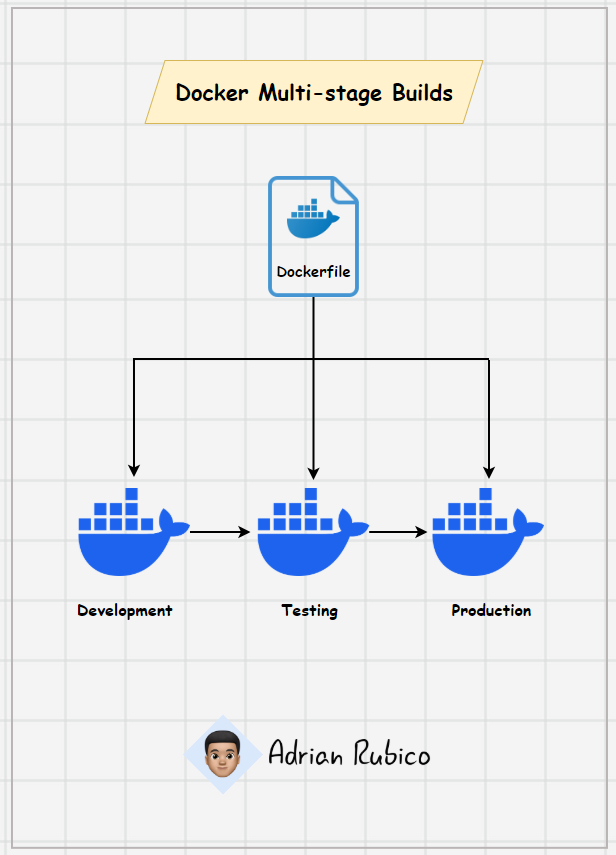
A multi-stage build is a technique in Docker that allows you to use multiple FROM
statements in a single Dockerfile. Each stage builds on the previous one, and only the necessary components are included in the final image.
Benefits of Multi-stage Build:
- Reduces Image Size – Removes unnecessary dependencies and build tools.
- Improves Security – The final image contains only runtime essentials.
- Enhances Performance – Speeds up the build process by caching dependencies.
Task: Implementing Multi-stage Build for DjangoCrudAjax
We will modify our previous Dockerfile and implement a multi-stage build.
Step 1: Modify the Dockerfile
In this step, we will modify our existing Dockerfile to use a multi-stage build. This approach will help reduce image size, improve security, and optimize performance by separating the build environment from the final runtime environment.
We will introduce two stages:
- Builder Stage: Installs dependencies and compiles assets.
- Final Stage: Copies only necessary files, keeping the final image lightweight.
# Stage 1: Build stage
FROM python:3.14-rc-slim AS builder
# Install system dependencies for MySQL client
RUN apt-get update && apt-get install -y \
default-libmysqlclient-dev \
build-essential \
pkg-config \
&& rm -rf /var/lib/apt/lists/*
# Set working directory
WORKDIR /app
# Set environment variables
ENV PYTHONDONTWRITEBYTECODE=1
ENV PYTHONUNBUFFERED=1
# Copy only necessary files for dependency installation
COPY requirements.txt ./
# Install dependencies
RUN pip install --no-cache-dir -r requirements.txt
# Stage 2: Final, lightweight runtime stage
FROM python:3.14-rc-slim
RUN apt-get update && apt-get install -y \
default-libmysqlclient-dev \
&& rm -rf /var/lib/apt/lists/*
# Set working directory
WORKDIR /app
# Copy application source code
COPY . /app/
# Copy installed dependencies from the builder stage
COPY --from=builder /usr/local/lib/python3.14/site-packages /usr/local/lib/python3.14/site-packages
COPY --from=builder /usr/local/bin /usr/local/bin
# Expose application port
EXPOSE 8000
# Entrypoint for executing commands
ENTRYPOINT ["sh", "-c"]
# Command to start the application
CMD ["python manage.py makemigrations && python manage.py migrate --noinput && python manage.py runserver 0.0.0.0:8000"]
By using multi-stage builds, we keep unnecessary build dependencies out of the final image, making it smaller, faster, and more secure.
Step 2: Build and Run the Multi-stage Docker Image
1️⃣ Build the Image
docker compose build
2️⃣ Compare the Image
- Before build

- After build

3️⃣ Run the Container
docker compose up -d
4️⃣ Check Running Containers
docker compose ps
5️⃣ Validate Application is Running
Visit http://localhost:8000
in your browser. 🚀
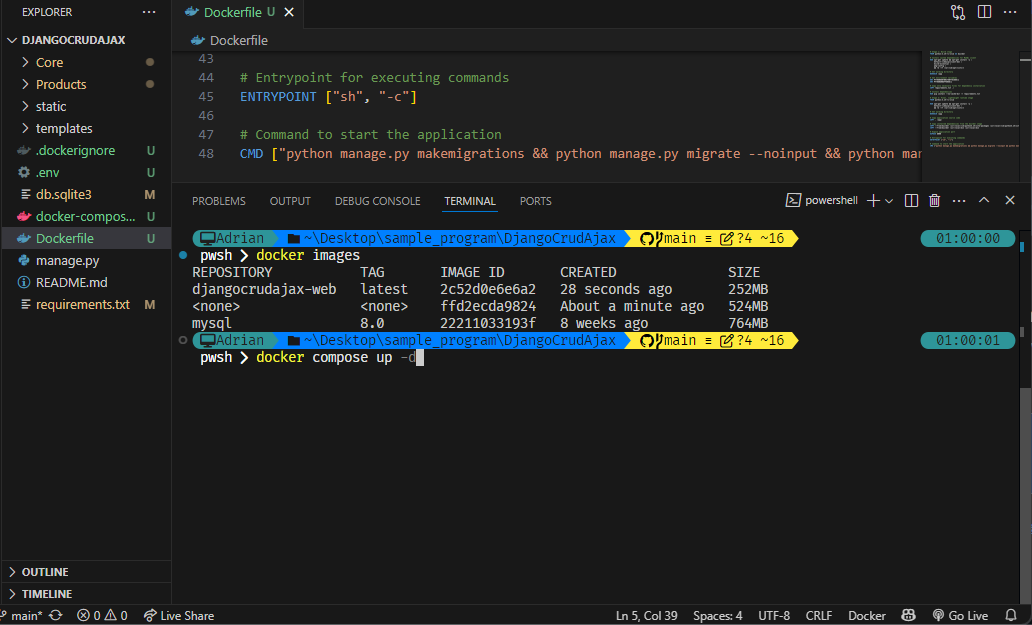
Conclusion
In this blog, we explored the multi-stage build process in Docker and how it helps optimize our container images. By separating the build environment from the runtime environment, we successfully reduced image size, improved security, and enhanced performance. This approach ensures that our final Docker image contains only the essential dependencies needed to run our Django application efficiently.
In the next blog, we will dive exploring Infrastructure as Code (IaC), its benefits, and how it simplifies cloud resource management with popular tools.
Day 25: Mastering Docker Multi-stage Builds
Learn how Docker multi-stage builds optimize images by reducing size, improving security, and enhancing performance for efficient deployments.
For the passion of automated cloud solutions.
Subscribe to get the latest posts. I mostly write about Backend (Python/Bash), DevOps and Linux.